Virtual JavaScript keyboard for HTML pages
How to add a virtual keyboard to your HTML pages. Excellent free solution to create touchscreen interfaces, but not only!
If you need to add a virtual keyboard to your HTML pages, I’ll explain how I did in simple steps.
A virtual keyboard (or virtual-keyboard ) is a graphical keyboard that appears on your computer screen allowing you to enter text (letters, numbers, symbols).
There are several types. I personally or try several, I think this one that I propose is the best.
In my specific case I needed a keyboard to use on a touch screen, with the following features
- che fosse personalizzabile, ovvero in cui i tasti da visualizzare fossero ben determinati
- che comparisse a schermo solo quando un campo di testo è selezionato (ha il focus)
I used this open-source project https://github.com/Mottie/Keyboard
of which you can see a demo here: https://mottie.github.io/Keyboard/
I’ll explain step by step how to integrate it into an HTML page.
If you are not interested in understanding step by step how to do it, go directly to the end of this article, you will find the downloadable package ready to use!
1.Prepare an HTML page
The first step is to create a blank HTML page.
Create an empty folder , name it “virtualkeyboard” and put a “ index.html ” file like this in it:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> </body> </html>
2. Download the Javascript library
Download the Mottie Virtual Keyboard project from here https://github.com/Mottie/Keyboard
and unzip it into your “virtualkeyboard” folder
You will get a second folder called “Keyboard-master” containing all the javascript and css files necessary for the keyboard to work.
3. Add JavaScript and CSS libraries
Add in section <HEAD> in the index.html file the link to:
- a jQuery library
- the virtual-keyboard library
- the necessary CSS
like this:
<!-- jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script> <!-- Virtual Keyboard --> <script src="keyboard-master/dist/js/jquery.keyboard.min.js"></script> <link rel="stylesheet" href="keyboard-master/css/keyboard-basic.css">
4. Add a text field
A keyboard is only useful if there is a space to type in, right?
Then create a text field and assign it the ID “ my-input-field “
Translated into code, insert the following code in the <BODY> tag:
<form action=""> <label for="my_input_field">Focus me:</label><input type="text" name="my_input_field" id="my_input_field"> </form>
5. Add JavaScript function to activate keyboard
Always in the <HEAD> tag insert this code:
<script> $(document).ready(function() { $.keyboard.keyaction.RETURN = function(base) { $('.ui-keyboard').blur(); do_something(); }; $('#my_input_field').keyboard({ layout: 'custom', // only use !! in the action key layout customLayout: { 'default': [ "1 2 3 4 5 6 7 8 9 0 - _ {bksp}", "Q W E R T Y U I O P /", 'A S D F G H J K L {RETURN}', "Z X C V B N M , .", "{space} @" ], }, display: { 'VIEW': 'Invio' }, usePreview: false, autoAccept: true, display: { 'bksp' : "\u2190" } }) // activate the typing extension .addTyping({ showTyping: true, delay: 250 }); }); function do_something() { alert("DO SOMETHING !"); } </script>
As you can see when loading jQuery libraries, the $ (document) function is triggered .ready
which calls the construction of the keyboard on the text field with ID = my_input_field through the function: $ (‘# my_input_field’). keyboard ({…. ..
Note that within this function, the customLayout property specifies all the keys that the keyboard must have, declared line by line.
A “custom” key called “RETURN” has also been declared which, if pressed, calls the do_something () function that will print an if, plice popup on screen.
The declaration of what the RETURN key should do when pressed is declared right at the beginning of the code, across the lines:
$.keyboard.keyaction.RETURN = function(base) { $('.ui-keyboard').blur(); do_something(); };
6. The complete code
In summary, the complete code for the index.html file is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <!-- jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script> <!-- Virtual Keyboard --> <script src="keyboard-master/dist/js/jquery.keyboard.min.js"></script> <link rel="stylesheet" href="keyboard-master/css/keyboard-basic.css"> <title>Document</title> <script> $( document ).ready(function() { $.keyboard.keyaction.RETURN = function(base) { $('.ui-keyboard').blur(); do_something(); }; $('#my_input_field').keyboard({ layout: 'custom', // only use !! in the action key layout customLayout: { 'default': [ "1 2 3 4 5 6 7 8 9 0 - _ {bksp}", "Q W E R T Y U I O P /", 'A S D F G H J K L {RETURN}', "Z X C V B N M , .", "{space} @" ], }, display: { 'VIEW': 'Invio' }, usePreview: false, autoAccept: true, display: { 'bksp' : "\u2190" } }) // activate the typing extension .addTyping({ showTyping: true, delay: 250 }); }); function do_something() { alert("DO SOMETHING !"); } </script> </head> <body> <form action=""> <label for="my_input_field">Focus me:</label><input type="text" name="my_input_field" id="my_input_field"> </form> </body> </html>
7. The final result
We’re done!
Now open the index.html file with a double click, the result is a blank page with an empty text field:

By clicking inside the field with the mouse or with your finger (if you use a touch monitor), the virtual keyboard will appear below
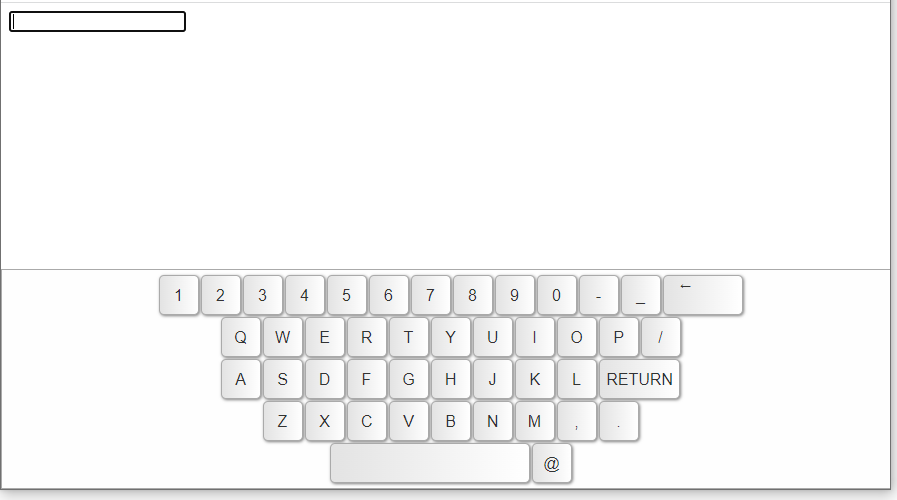
At this point you can type your text by clicking on the buttons corresponding to the letters, either with the mouse or with your finger (if you have a touchscreen).
Clicking on the RETURN key activates the “do_something” function which causes an alert “DO SOMETHING!”
If you remove the focus from the field, the keyboard disappears.
8. Improvements
For my specific project the keyboard was too small, as I use large screens.
I then created a second CSS file (alternative to the default one) by increasing the size of the keys. I called this file “keyboard-master/css/keyboard-basic-custom.css” and you can find it in the downloadable package at the end of this article.
In the package you will also find the STANDARD CSS file: “keyboard-master/css/keyboard-basic.css”
You choose the one to use!
If you want to have bigger keys use this file:
<link rel="stylesheet" href="keyboard-master/css/keyboard-basic-custom.css">
If you want to use the default CSS file of the “virtual-keyboard” package, use this file:
<link rel="stylesheet" href="keyboard-master/css/keyboard-basic.css">
9. Download the full package
If you are interested in trying the example code right away, download the full package here.
10. Other examples of configurations
If you want to try other examples of configurations, I refer you to this page: https://mottie.github.io/Keyboard/
Help me support this blog
If you want you can help me concretely to support this blog. You can do it with a free donation, by credit card or paypal. I will be grateful to you.
Несомненно важные новости индустрии.
Важные события мировых подуимов.
Модные дома, торговые марки, гедонизм.
Лучшее место для модных хайпбистов.
https://donnafashion.ru/
Наиболее стильные новинки индустрии.
Абсолютно все мероприятия самых влиятельных подуимов.
Модные дома, бренды, гедонизм.
Лучшее место для трендовых хайпбистов.
https://donnafashion.ru/
Очень актуальные события подиума.
Все эвенты самых влиятельных подуимов.
Модные дома, лейблы, высокая мода.
Интересное место для модных людей.
https://mvmedia.ru/novosti/282-vybiraem-puhovik-herno-podrobnyy-gayd/